Contact Fields Mapping Configuration
Describes how to configure contact field mapping options for the Claspo Editor.
Overview
Contact fields mapping allows users to connect form fields with their contact management system. When properly configured, the editor provides a UI for mapping form inputs to contact database fields.
UI Representation
The contact mapping functionality will appear in the editor as dropdown options when user drops new control to the Editor. Form fields can be mapped to contact fields
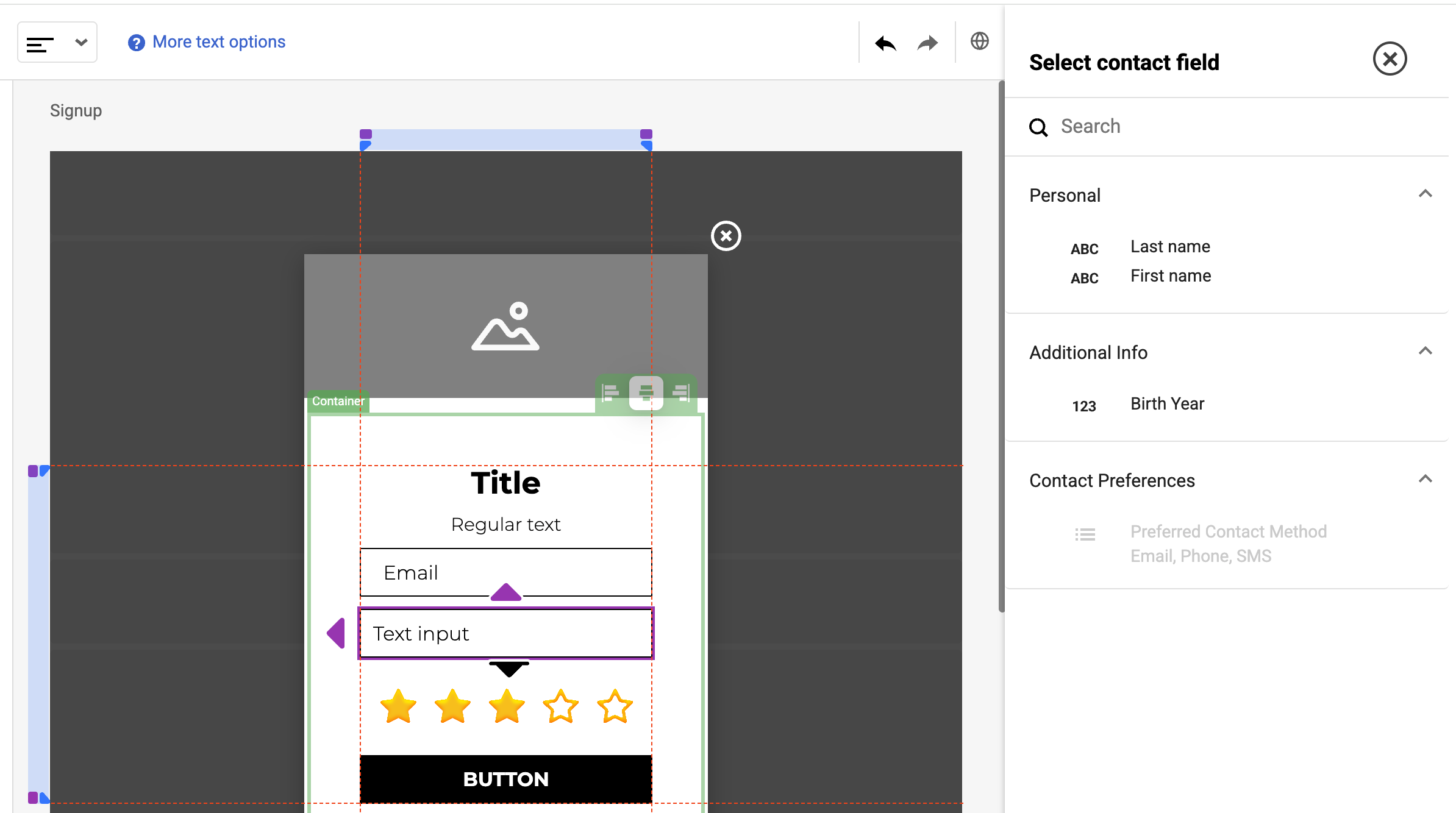
The groups specified in the group
property will be displayed as collapsible sections in the UI, allowing for organized selection of fields.
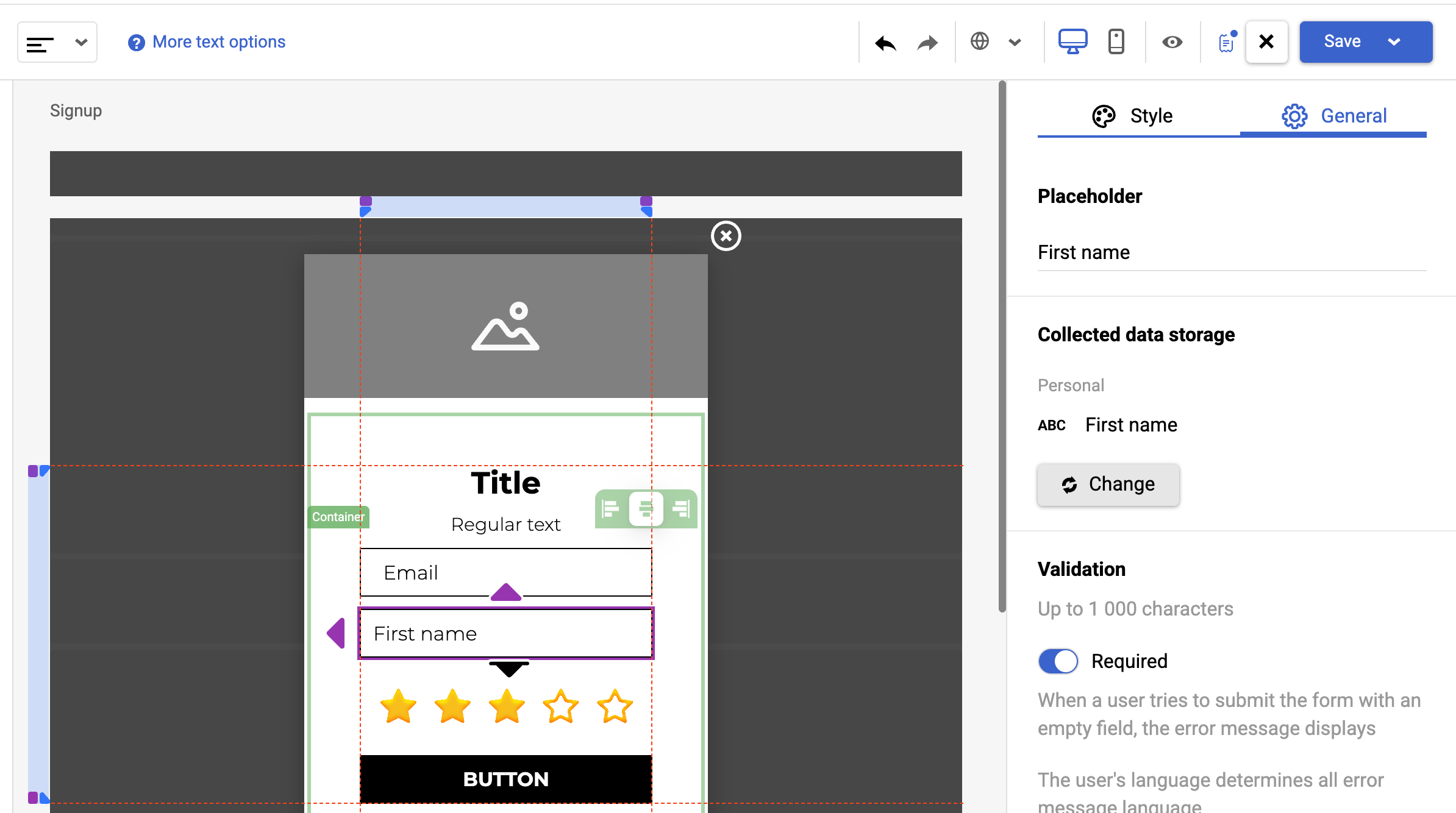
Field's name
property will appear as input placeholder (if applicable to component) at the editor. A key of id
property will be written to the CONTACT_DATA_SUBMIT
event on the script side
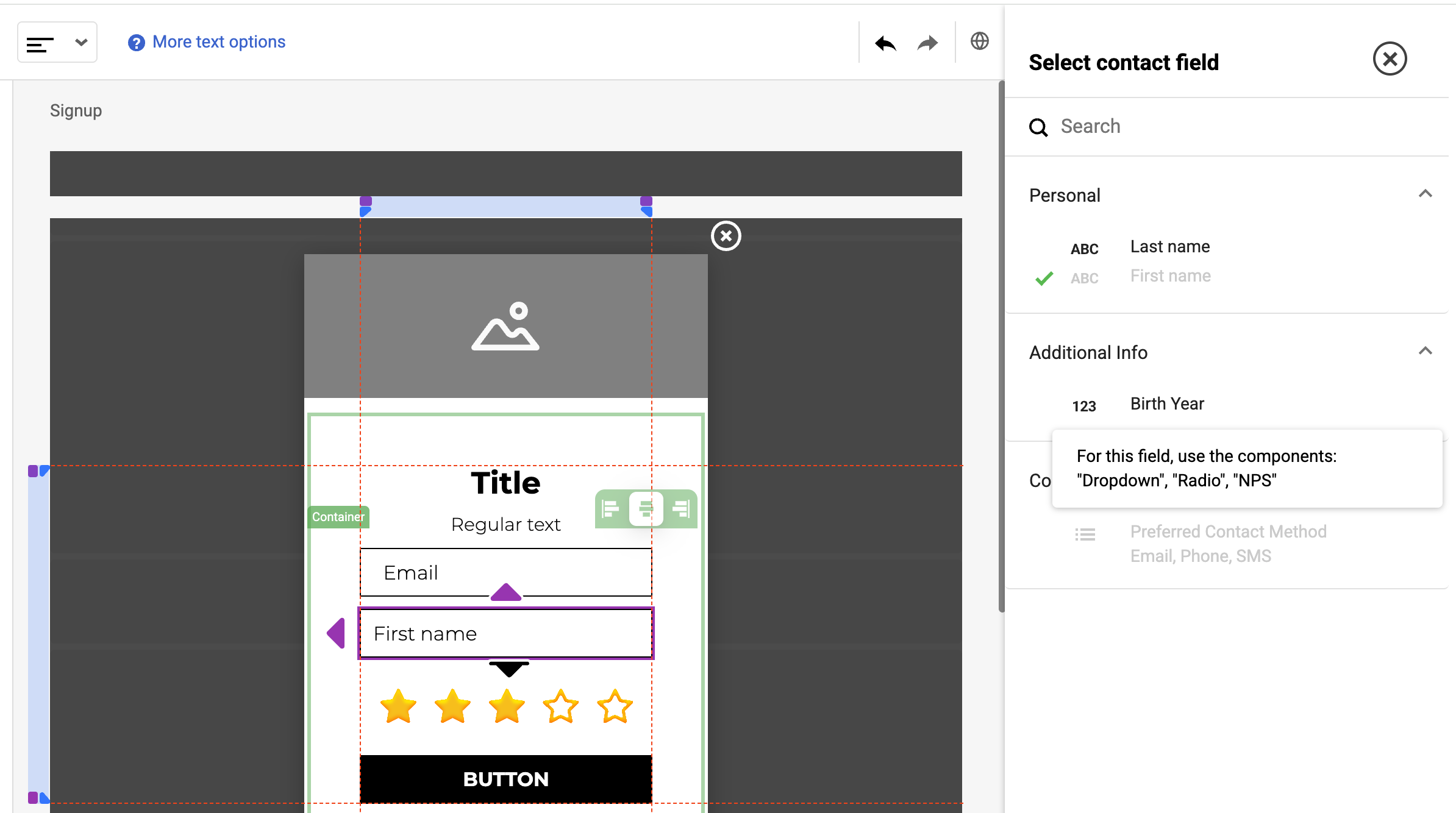
If the field type is not matched with the control type then it will be disabled from the selection.
Configuration Requirements
To enable contact fields functionality in the editor:
- Set
useContactFields: true
in the main editor configuration Editor Configuration - Implement the required contact mapping API methods Editor HTTP API Configuration
API Methods
The following methods need to be implemented in your AppApiConfigI
implementation:
getContactMappingOptions
This method should return a list of available contact fields that users can map their form fields to.
getContactMappingOptions: () => Promise<ContactMappingOptionI[]>;
ContactMappingOptionI Interface
interface ContactMappingOptionI {
id: string; // Unique identifier. A key of the form that will be written to the `CONTACT_DATA_SUBMIT` event on the script side
name: string; // Display (placeholder) name at the editor
group: string | null; // Group name the field belongs to, on the UI groups are collapsed menu
type?: ContactMappingOptionType; // Field type
values?: string[]; // Possible values for select type
options?: ContactMappingOptionLimitsI; // Configuration options
}
ContactMappingOptionType Enum
enum ContactMappingOptionType {
TEXT = 'text',
SELECT = 'select',
CHECKBOX = 'checkbox',
TEXT_AREA = 'textarea',
NUMBER = 'number',
DATE = 'date',
DATE_TIME = 'datetime',
BOOL = 'bool',
DECIMAL = 'decimal',
UNKNOWN = 'unknown'
}
Example
import { AppConfigI } from '@claspo-editor';
// Step 1: Define your contact mapping service
export class ContactMappingService {
// Get available contact mapping options
getContactMappingOptions(): Promise<ContactMappingOptionI[]> {
// Fetch from your API or use static data
return Promise.resolve([
{
"id": "last_name",
"name": "Last name",
"group": "MAIN",
"type": "text",
"values": []
},
{
"id": "first_name",
"name": "First name",
"group": "MAIN",
"type": "text",
"values": []
},
{
"id": "birth_year",
"name": "Birth Year",
"group": "Additional Info",
"type": "number",
"values": []
},
{
"id": "preferred_contact_method",
"name": "Preferred Contact Method",
"group": "Contact Preferences",
"type": "select",
"values": [
"Email",
"Phone",
"SMS"
]
}
]);
}
// Optional methods for creating custom fields
getContactFieldGroups() {
return Promise.resolve([]);
}
createContactFieldsGroup(params) {
return Promise.resolve({});
}
createContactField(params) {
return Promise.resolve({});
}
}
// Step 2: Create service instance
const contactMappingService = new ContactMappingService();
// Step 3: Configure the editor with contact fields support
const editorConfig: AppConfigI = {
containerElement: document.getElementById('editor-container'),
countryCode: 'US',
theme: {
primaryColor: '#3f51b5',
secondaryColor: '#f50057',
// ... other theme options
},
notificationHandlers: {
success: msg => console.log('Success:', msg),
info: msg => console.log('Info:', msg),
warning: msg => console.warn('Warning:', msg),
error: msg => console.error('Error:', msg),
hide: () => console.log('Notifications hidden')
},
closeEditorCallback: (variant) => console.log('Editor closed'),
afterSavedCallback: (variant) => console.log('Widget saved'),
staticResourcesUrl: 'https://static.example.com',
hostUrl: 'https://app.example.com',
// Enable contact fields
useContactFields: true,
// Configure API
api: {
// Widget operations
getWidgetAppearances: (widgetId, variantId) => {
return fetch(`/api/widgets/${widgetId}/variants/${variantId}/appearances`)
.then(response => response.json());
},
// Contact mapping operations
getContactMappingOptions: contactMappingService.getContactMappingOptions,
// Other required API methods
// ...
}
};
// Step 4: Initialize the editor
initEditor(editorConfig);
Testing Contact Fields Mapping
To test your implementation:
- Configure the editor with
useContactFields: true
- Implement the required contact mapping API methods
- Open editor and drop input component
- Verify that your contact fields appear in the mapping dropdown
- Map fields to contact fields
- On the script side, fill the form and verify that the correct data is sent to your contact system
Updated 5 days ago